5 javascript shorthands you should start using today - part 1
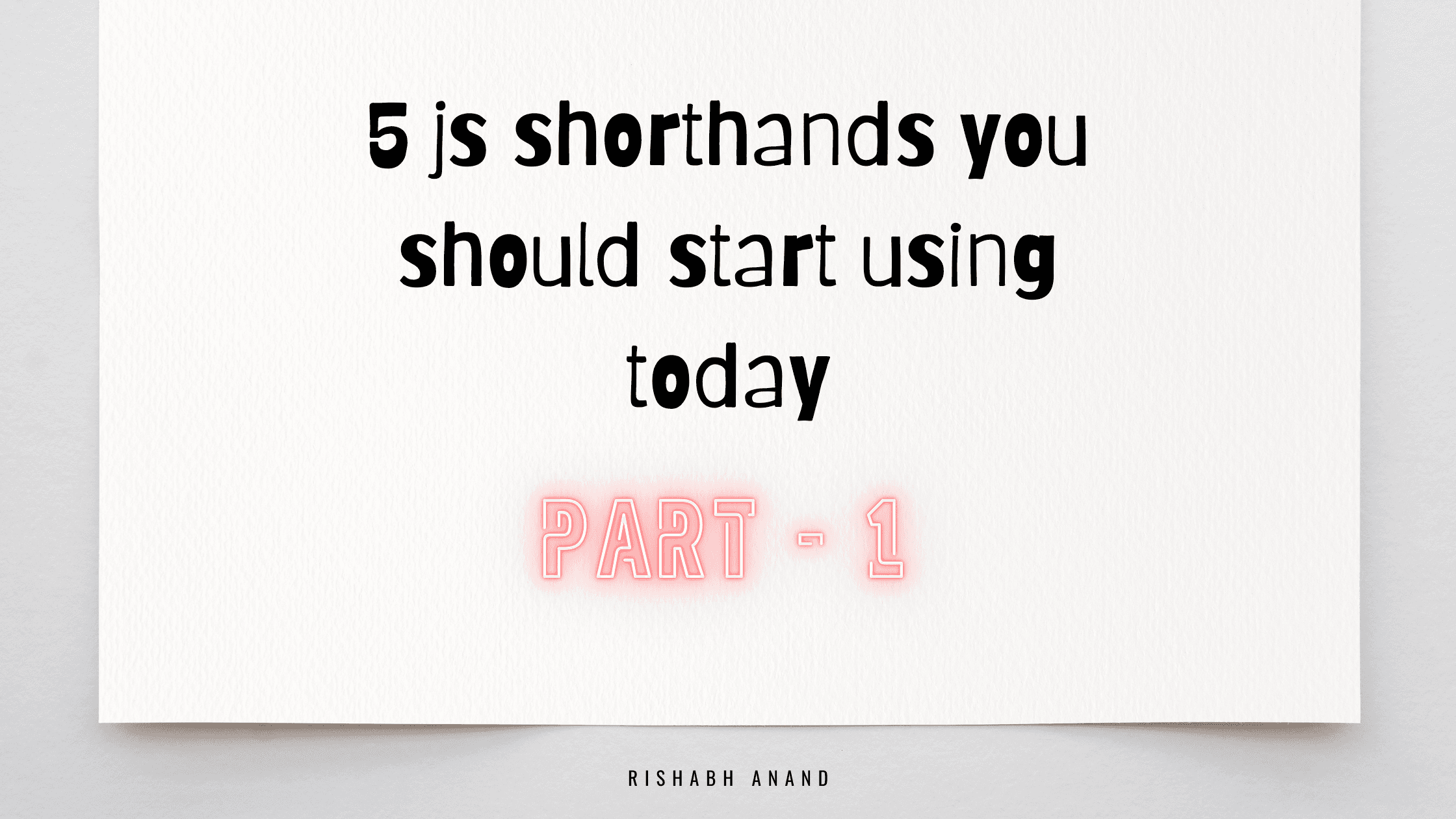
5 Javascript shorthands you should start using today - part 1
1. Make use of Truthy value
In javascript you have an option to remove equality operator by using the truthy values in relevant conditions.
Longhand ❌:
if (variable === true) {
// code goes here
}
If the value of variable
here is any of the truthy values, we need not use the quality operator at all.
Shorthand ✅:
if (variable) {
// code goes here
}
Please refer this link for all truthy values: All Truthy values
2. Short-circuit logical operator
When we could do something in just a line using logical operator why waste n lines for same.
Longest ❌:
let port;
if (process.env.PORT) {
port = process.env.PORT;
} else {
port = 3000;
}
Long ❌:
let port = 3000;
if (process.env.PORT) {
port = process.env.PORT;
}
Shorthand ✅:
const port = process.env.PORT || 3000;
3. Arrow functions
Longhand ❌:
function helloWorld(name) {
console.log("Hello", name);
}
setTimeout(function () {
console.log("Loaded");
}, 2000);
names.forEach(function (name) {
console.log(name);
});
Shorthand ✅:
helloWorld = (name) => console.log("Hello", name);
setTimeout(() => console.log("Loaded"), 2000);
names.forEach((name) => console.log(name));
4. Spread Operator Shorthand
Longhand ❌:
// Shallow merge objects
const name = { "first": "Virat", "last": "Kohli" };
const addr = { "floor": "4th", "street": "Chaploon street" };
const details = Object.assign({}, name, addr, ...);
// joining 2 arrays
const fibo1 = [1, 1, 2, 3];
const nacci1 = [5, 8, 13].concat(fibo1);
// cloning 2 arrays
const fibo2 = [1, 1, 2, 3];
const nacci2 = [5, 8, 13].slice()
Shorthand ✅:
// joining 2 arrays
const fibo1 = [1, 1, 2, 3];
const nacci1 = [...fibo1, 5, 8, 13];
console.log(nacci1); // [ 2, 4, 6, 1, 3, 5 ]
// cloning 2 arrays
const arr = [1, 2, 3, 4];
const arr2 = [...arr];
5. The mighty Ternary operator
Why write multiple lines of conditional code if you could just do it in one line using ternary operators.
Longhand ❌:
let info;
if (value < 10) {
info = "Value is too small";
} else if (value > 20) {
info = "Value is too large";
} else {
info = "Value is in range";
}
A concise if-else
condition
Shorthand ✅:
const info =
value < 10
? "Value is too small"
: value > 20
? "Value is too large"
: "Value is in range";
Beatified Shorthand ✅✅:
const info =
value < 10
? "Value is too small"
: value > 20
? "Value is too large"
: "Value is in range";
But many times we have to call functions in if-else conditions, what do we do there?
Longhand ❌:
if (condition) {
f1();
} else {
f2();
}
Shorthand ✅:
(condition ? f1 : f2)(); // this is a way that can fetch you a offer letter in an interview.
Check the same code here Codesandbox
That was all folks 😎! We will continue with some more interesting shorthands and tricks in the next one.
Do share this with your friends and colleagues.
Want to see what I am working on? Github
Want to connect? Reach out to me on Linkedin
Follow me on Instagram to check out what I am up to.